Given a square matrix, return the sum of the matrix diagonals.
Only include the sum of all the elements on the primary diagonal and all the elements on the secondary diagonal that are not part of the primary diagonal.
Example 1:
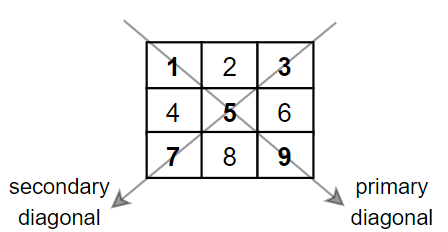
Input: mat = [[1,2,3], [4,5,6], [7,8,9]] Output: 25 Explanation: Diagonals sum: 1 + 5 + 9 + 3 + 7 = 25 Notice that element mat[1][1] = 5 is counted only once.
Example 2:
Input: mat = [[1,1,1,1], [1,1,1,1], [1,1,1,1], [1,1,1,1]] Output: 8
Example 3:
Input: mat = [[5]] Output: 5
Constraints:
n == mat.length == mat[i].length
1 <= n <= 100
1 <= mat[i][j] <= 100
Solution:
public int DiagonalSum(int[][] mat) {
int sum = 0;
int rowS=0,colE=mat[0].Length-1;
for (int i = 0; i < mat.Length; i++)
{
for (int j = 0; j < mat[i].Length; j++)
{
if(i==j || (rowS==i && colE==j))
{
sum+=mat[i][j];
}
}
rowS++;
colE--;
}
return sum;
}
Explanation :
Diagonal left to right, the value of i
is equal to the value of j
. mat[0,0],mat[1,1],mat[2,2] all are left diagonal element but we in sum we need diagonal element from right to left so in this case we need to check the values of i
and j
.
For elements 3, 5, and 7, the values of i, j
are i=0,j=2
,i=1,j=1
and i=2,j=0
, here observation is i
value increasing and j
value is decreasing. We are using rowS,colE
these variables in if condition
and after execution of the internal for loop we are incrementing(rowS) and decrementing(colE).
Time Complexity:
O(N2)
Need help?
Read this post again, if you have any confusion, or else add your questions to Community